My Projects
Linear Programming
Linear programming is an optimization technique used to determine the best possible outcome, such as maximizing profit or minimizing cost, within a given set of constraints. It involves formulating a mathematical model where the objective function, representing the goal (e.g., profit or cost), is linear, and the constraints, which limit the resources or conditions (e.g., budget, time, materials), are also expressed as linear inequalities or equations. The purpose of linear programming is to identify the optimal solution that satisfies all constraints while achieving the desired maximum or minimum value of the objective function.
I applied linear programming to solve a Sudoku puzzle by modeling the Sudoku rules as linear constraints. This allowed me to set up a system where the goal was to fill the Sudoku grid while ensuring that each row, column, and 3x3 region contained unique numbers from 1 to 9.
Python Data Analytics
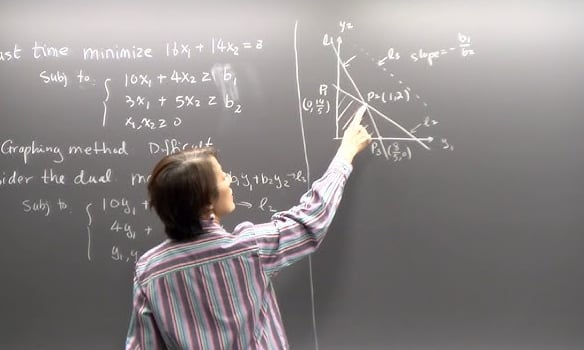
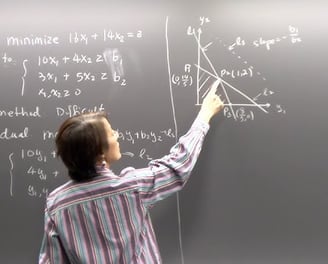
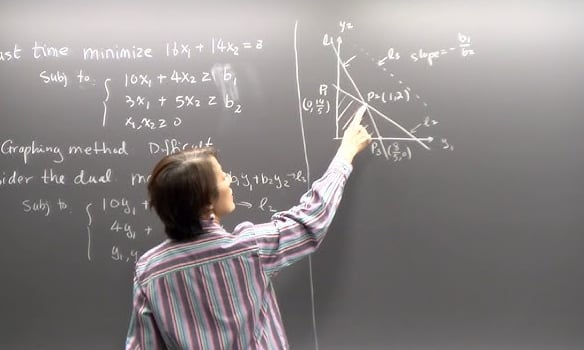
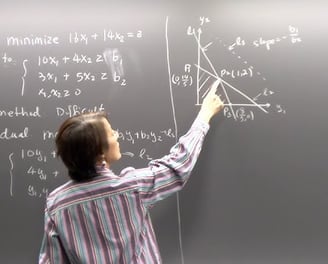
Linear Programming II
Linear programming is an optimization technique used to determine the best possible outcome, such as maximizing profit or minimizing cost, within a given set of constraints. It involves formulating a mathematical model where the objective function, representing the goal (e.g., profit or cost), is linear, and the constraints, which limit the resources or conditions (e.g., budget, time, materials), are also expressed as linear inequalities or equations. The purpose of linear programming is to identify the optimal solution that satisfies all constraints while achieving the desired maximum or minimum value of the objective function.
I used linear programming to optimize a factory's production of small and large units. By modeling production costs, resource limits, and storage constraints, I determined the most cost-effective way to meet monthly demand while staying within resource and space limits.
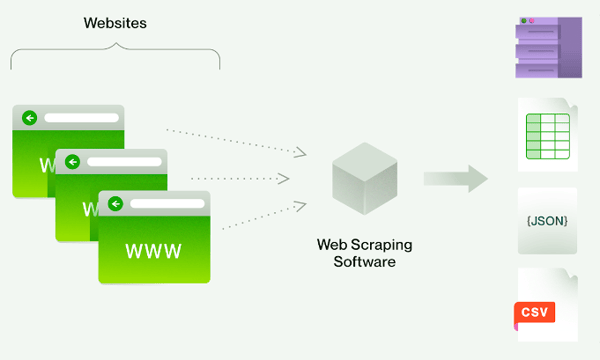
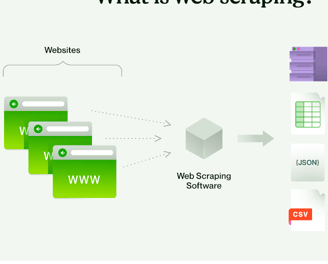
Web Scraping
Web scraping is the process of using a computer program to automatically collect information from websites. Instead of manually copying and pasting data from a webpage, web scraping allows you to quickly gather large amounts of data by having a program visit the website, find the information you need, and save it to a file or database. It's like sending a robot to browse the internet for you and bring back the data you're interested in.
This script functions as a web scraper that extracts data from a specific webpage and saves it into a CSV file. It begins by importing necessary libraries for HTTP requests, HTML parsing, and data handling, and sets up parameters for the URL and file paths. The script sends a GET request to the webpage, parses the HTML content, and locates a specific div element containing the data of interest, such as a scoreboard. It then iterates through each table row (tr element) within this div, checking for valid rows with the required number of table data (td) elements. For each valid row, it extracts a link to a detailed user page, makes another request to retrieve this detailed information, and writes the extracted data (including user names, ranks, and other metrics) into a CSV file. The script also includes a random delay between processing each row to mimic human behavior and avoid server overload, thereby reducing the likelihood of being blocked by the server.
Java CS
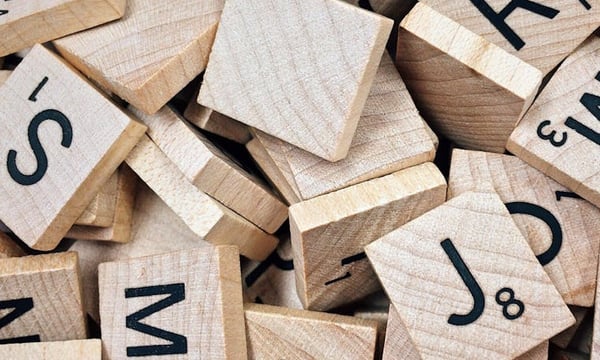
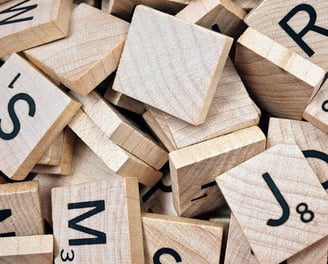
Wordle Game
This project is about a Wordle game using Java, which replicates the popular word-guessing game. The project includes several key components:
Game Logic: The WordleGame class encapsulates the core logic, managing the state of the game, including the user's guesses, the current puzzle number, and the solution word. It handles the evaluation of each guess, comparing it to the correct answer and assigning appropriate colors (green, yellow, red) to indicate correct positions, correct letters in wrong positions, or incorrect letters.
User Interface Representation: The WordleLetter class models individual letters in a guess, with color-coding to reflect their correctness. This class includes methods for setting and getting the letter's color, and a toString method that integrates color codes into the console output, giving a visual representation of the game state.
Word Bank Management: The WordBank class provides functionality to fetch the correct answer for a given puzzle number and to validate user guesses against a dictionary of valid words. This ensures that all guesses are legitimate and keeps the game challenging.